Something my language does differently from most object-oriented languages is that there’s no implicit this value for member functions. This may seem strange at first, but it solves a few problems.
A common problem with an implicit this value is that the function must be called explicitly as a member function to receive the this value. For example, in JavaScript, calling object.function() passes object into function as this. One case where this goes wrong is if you want to use that function as a delegate: control.onClick = object.function. Now, when control.onClick() is called, it will pass control to function as this instead of object. This is sometimes useful, but it’s unintuitive behavior for a function originally declared as part of object. JavaScript provides a way to override the this pointer passed to the function, but the fact that this may not be the object the function was declared in is a caveat most JavaScript programmers will have to deal with.
The next level of this problem is that when you have nested object declarations, this only allows you to access the innermost object’s members. That sounds a little contrived, but it’s a real problem. For example, if you have an object for your module’s public members, and within it you have another object literal, that nested object’s member definitions cannot access the module’s public members.
This is actually a little better in JavaScript than in languages like C++, since each object has its own map from member names to values. Since a function value in JavaScript includes an implicit lexical closure, the member function for an object can have implicit information about its lexical environment. That normally doesn’t include this, but it allows CoffeeScript to generate JavaScript that binds a function value to a specific value of this, so it will be the same regardless of how the function is called. However, that solution still requires programmers to understand how JavaScript defines this, so they can decide which functions need the special this-binding function syntax, and it doesn’t solve the problems with nesting objects. For more info, see CoffeeScript’s function binding.
The way I solved this in my language is to replace the caller-provided this with capturing the object’s members as part of a lexical closure. Object members may be accessed within the scope of the object definition, and it will resolve to the inner-most object that defines the name. Internally, there isn’t a single this, and member names need to be resolved against a stack of objects that were in the lexical scope.
To make things a little bit clearer for everybody (humans and machines), member names must start with a . symbol, both when declared and when accessed. Objects may also include definitions that don’t have a . prefix, but they are the equivalent of private members: they cannot be accessed outside the object definition. When an object is used as a prototype, its public and private members are copied into the derived object, and are bound to the new object where they previously accessed the prototype’s public members.
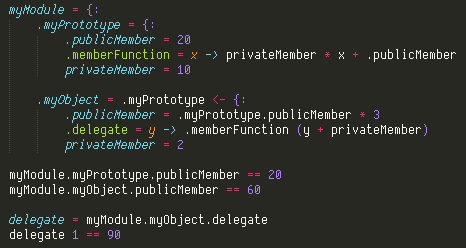
Member name resolution in my language
There are still some remaining problems:
- There aren’t aliases for the objects that are being used to resolve member names. This is rarely needed, but it is occasionally useful. The simplest solution would be to define this(or .) to be the innermost object context, but that doesn’t allow e.g. memberFunction to access the module object it is being used from; it can’t simply refer to myModule, as myModule may be used as a prototype for another object, and if that is how memberFunction was accessed, then that prototyped object is what you want.
- I’m torn on using a symbolic <- for object prototyping. Its meaning is similar to class inheritance, where the left operand is a prototype object for the right operand. An operator is more flexible than making the prototype part of the object literal syntax, and <- makes the non-commutative nature of the operator clear, but the meaning isn’t as obvious to people who don’t know the language as using a keyword like extends would be. But what’s an appropriate keyword that keeps the prototype as the left operand?
- The way I implement this when running in the JavaScript VM, objects include an implicit function that is called when it is used as a prototype. The function recreates the prototype’s member definitions with the new object bound in place of the prototype object. This function implicitly includes a closure for the lexical scope the object was created in, and if the prototype object is itself created from a prototype, a call to that prototype’s prototyping function. The problem is that a VM may keep the whole lexical scope around, instead of just the variables accessed by the functions; the JavaScript VM I am using (V8) is implemented that way. As a result, any definitions accessible from an object’s scope are kept around as long as the object is, since they may be accessed when the object is used as a prototype. The compiler has the information it needs to avoid this when it is unnecessary, but I don’t like to rely on that kind of optimization for intuitive performance/memory characteristics.